Trainings and Consulting
С++ - is huge. Trainings and Consulting are meant for helping you to deal with the language, show best practices and improve quality of your applications.
I'd be pleased to answer any questions you may have. Use e-mail for contacting me: antoshkka@gmail.com (note the two 'k'!)
Courses on any C++ related topics could be done on demand.
From C into С++
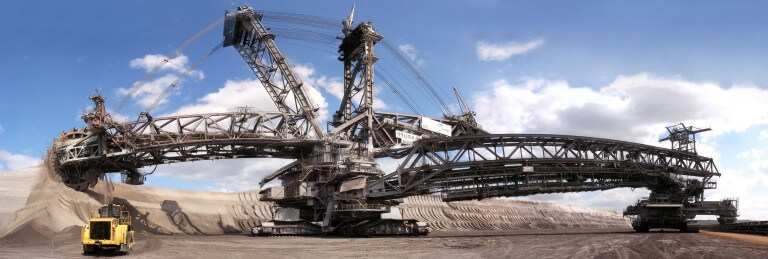
Practical course for people with knowledge of C or basic knowledge of C++, wishing to learn or improve their C++:
- Intro:
- C code and common problems
- Code in С++
- RAII and destructors
- Language basics
- references and const references
- variables
- functions
- function arguments
- returning parameters from functions (simplified)
- function overloading
- ostreaming values
- Classes (basics 1)
- class variable
- member initialization
- private/public
- member functions and operators
- constant member functions and operators
- default constructor
- operator overloading
- Classes (basics 2)
- copy constructor
- move constructor
- =deleted;/=default;
- destructor
- explicit conversion operator
- std::string
- examples
- performance
- range based for
- ostreaming
- std::vector
- examples
- initilizer list
- performance and pitfalls
- ostreaming
- auto
- iterators
- Lambdas
- "functions without a name"
- capture
- capture expressions (C++14)
- mutable
- Algorithms and Iterators
- Big-O
- Big-O in practice
- functional object
- algorithms for everyday use
- pitfalls of the <algorithm> (memory allocations)
- Classes (inheritance)
- private inheritance
- protected
- public inheritance + protected destructor
- slicing
- static functions
- static variables
- ostream operator
- Classes (polymorphism)
- pure virtual functions
- virtual functions
- final/override
- virtual destructor
- static_cast, dynamic_cast, const_cast, reinterpret_cast
- Error handling
- exceptions
- noexcept
- std::error_code
- Combining approaches
- Templates
- Template functions
- Template classes
- template aliases
- Template member functions
- <type_traits> and static_assert
- Containers
- std::string
- std::vector
- std::unordered_map/set
- std::map/set
- heterogenous lookup
- std::deque
- std::list
- std::forward_list
- C++11 and C++14
- std::move
- rvalue & lvalue
- forwarding/universal reference
- returning values from functions
- move-only data types
- move_iterator
- "pinned" data types
- unique_ptr
- shared_ptr and make_shared
- pair
- tuple
- constepxr
- Multithreading
- thread (detach, join)
- mutex + lock
- atomic
- thread_local
- memory models
- hardware destructive size + alignas
- pitfalls (multiple locks, RAII)
- magic statics + call_once
- shared_mutex (C++17)
- Queues
- conditional_variable (effective notify())
- function
Effective usage of the Standard Library and Boost
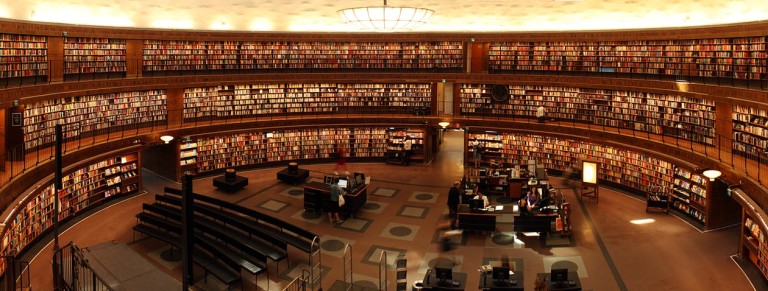
Course is devoted to effective solving of tasks using Standard Library and Boost. Topics:
- Algorithms:
- Big-O
- Big-O in practice
- Algorithms for everyday use that you've missed
- Pitfalls of the <algorithm> (memory allocations, move semantics and iterators)
- Data structures:
- string (SSO, empty strings, cache friendliness)
- string_view (empty strings, 0-termination, cache friendliness)
- vector (allocations, cache friendliness)
- list/deque (allocations, multithreading)
- unordered_map (perf, limitations, more effective solutions)
- map
- Other vectors (small, stack)
- Flat associative containers
- Pitfalls (noexcept, move construction)
- Optimization tips (move, move_iterator)
- Multithreading:
- thread (detach, join)
- mutex
- atomic (memory model)
- conditional_variable (effective notify())
- call_once
- Pitfalls (multiple locks, RAII)
- Optimization tips (critical section size, performance in case of cores growth)
- For everyday coding:
- variant
- optional
- unique_ptr
- shared_ptr
- ProgramOptions
- Filesystem
- LexicalCast
- function
C++11, C++14 and C++17
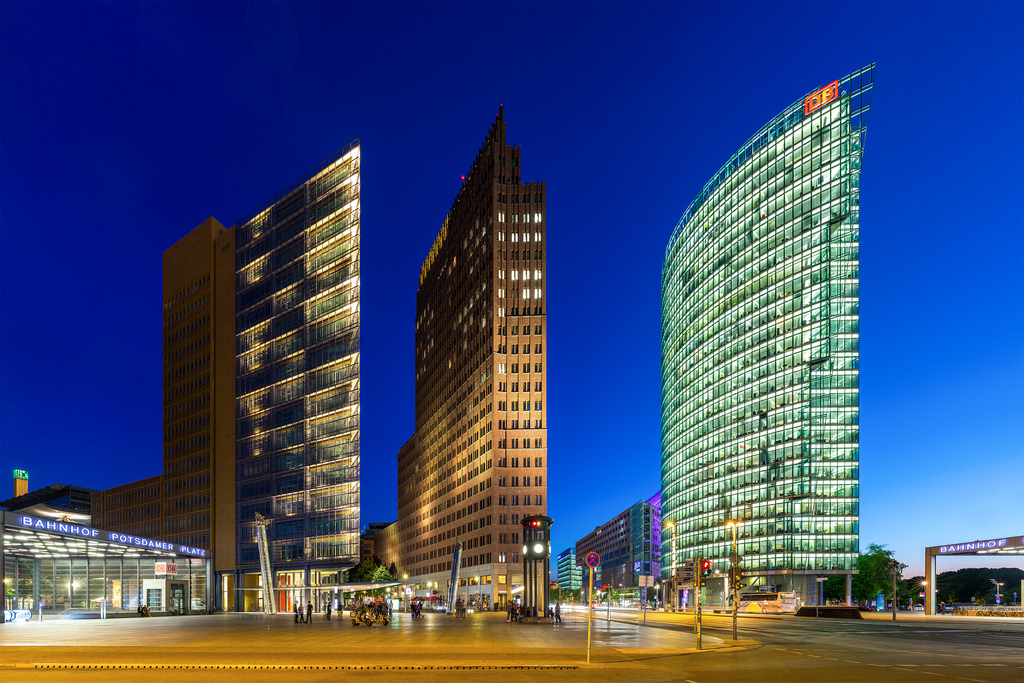
Course is devoted to effective solving of tasks using modern features of C++. Topics:
- C++11:
- move semantics
- auto
- range based for
- initilizer list
- lambda
- final/override
- member initialization
- static_assert
- noexcept
- constexpr
- =default/=delete
- unordered_map
- tuple
- unique_ptr
- shared_ptr
- function
- Random numbers
- thread (detach, join)
- mutex
- atomic (memory model)
- conditional_variable (effective notify())
- call_once
- C++14:
- generic lambda
- lambda capture expressions
- [[deprecated]]
- shared_mutex
- Heterogeneous search
- C++17:
- string_view
- optional
- variant
- filesystem
- any
- nodes
- byte
- parallel algorithms
- special math
- structured bindings
- if constexpr
- if/switch with initialization
- deduction guides
- Migration to the modern Standards:
- C++?? -> C++11
- C++?? -> C++14
- C++?? -> C++17
- What Standard do we have to use right now??
- Performance
- Development speed
- Labor costs